Robot Operating System (ROS)
What is ROS
ROS is a comprehensive collection of libraries, frameworks, and tools designed to facilitate the development and operation of various types of robots. It provides a flexible and modular platform that enables seamless integration of different software components and hardware devices, allowing for efficient robot control and communication.
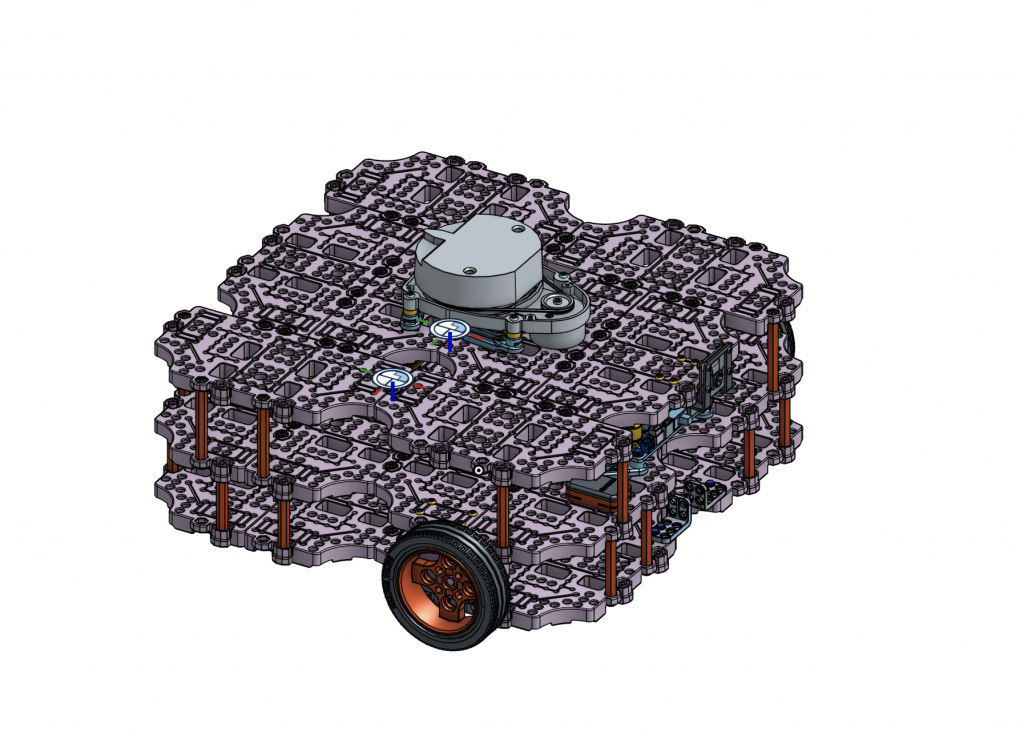
How does it work?
ROS (Robot Operating System) utilizes a Subscriber model for controlling robots. Publishers transmit information, while subscribers listen to the published information, which is referred to as a Topic. Topics can cover a range of data, including position information and command instructions. This communication model allows different components of a robot system to exchange data effectively and coordinate their actions. It promotes modularity, scalability, and reusability in developing complex robotic applications.
Getting Started
To start working with ROS, you’ll need to have it installed on your system. ROS supports different versions, but for the purpose of this tutorial, we’ll focus on ROS Melodic, which is compatible with Ubuntu 18.04. You can install ROS Melodic by following the official installation instructions provided on the ROS wiki (ros.org). Be sure to install the version of ROS that works with your operating system.
Once ROS is installed, you can begin creating your ROS workspace. A workspace is a directory where you’ll organize your ROS packages. Packages are the fundamental building blocks of a ROS system and contain libraries, executables, scripts, and other resources. To create a new workspace, open a terminal and enter the following commands:
$ mkdir -p ~/catkin_ws/src $ cd ~/catkin_ws/ $ catkin_make
Next, let’s create a simple ROS package. Packages provide a modular structure to your ROS project and encapsulate related functionality. In the terminal, navigate to your workspace’s source directory:
$ cd ~/catkin_ws/src $ catkin_create_pkg my_package rospy std_msgs
This command creates a package named “my_package” that depends on the “rospy” and “std_msgs” packages. “rospy” is the ROS Python library, and “std_msgs” provides standard message types used in ROS.
Adding a ROS Node
Now, let’s create a ROS node within our package. Nodes are individual programs that perform specific tasks and communicate with each other using topics, services, and actions. Create a new Python script called “my_node.py” within the “scripts” directory of your package:
$ cd ~/catkin_ws/src/my_package/scripts $ touch my_node.py $ chmod +x my_node.py
Open “my_node.py” in a text editor and add the following code
#!/usr/bin/env python import rospy from std_msgs.msg import String def callback(data): rospy.loginfo("Received: %s", data.data) def my_node(): rospy.init_node('my_node', anonymous=True) rospy.Subscriber('my_topic', String, callback) rospy.spin() if __name__ == '__main__': my_node()
We import the necessary ROS libraries in this example, including the “String” message type from “std_msgs.” We define a callback function that will be executed whenever new data is received on the “my_topic” topic. The “my_node” function initializes the ROS node, sets up the subscriber, and enters a loop to keep the node running.
Building And Run
To build your package, go back to the root of your workspace and run
$ cd ~/catkin_ws $ catkin_make
Assuming there are no errors, you can now run your ROS node. Open a new terminal and execute the following command:
$ source ~/catkin_ws/devel/setup.bash $ rosrun my_package my_node.py
Your node is now running and waiting for messages on the “my_topic” topic. To send messages to the topic, you can use the rostopic
command-line tool. Open another terminal and execute the following command:
$ source ~/catkin_ws/devel/setup.bash $ rostopic pub my_topic std_msgs/String "data: 'Hello, ROS!'"
You should see the message “Received: Hello, ROS!” displayed in the terminal where your node is running.